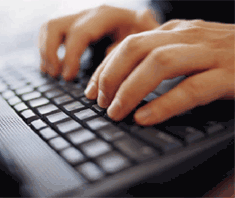
How to Use Type-Ins Effectively
Last updated: Saturday July 13th, 2019
On JetPunk, we have what's called type-ins. These use regular expressions to pattern-match what the user has entered into the text box against set expressions. For example, the expression might simply mean "type DOG exactly", so the user would have to type in the word "dog".
At this point it would be good to mention that JetPunk is not case sensitive, meaning that "AlpHa" is considered the same as "aLPHa". This is useful since we don't have to distinguish between capital letters and lowercase.
When creating type-ins, for many answers an automatic type-in will be loaded by default. This is mainly for common answers like Countries, Cities or States, though there are many others on there also. If it is not one of these common answers, then JetPunk will simply suggest a type-in base on the letters, such as a "y" at the end of a word will have the type-in "(EE|EY|IE|Y)" suggested. We'll go through what that means later.
Simple Type-Ins
There are many simple ways to add type-ins to JetPunk answers. For each answer, you are presented with three options: "Auto", "Custom" or "Exact".
- Auto will use the automatic type-in if available or the suggested type-in otherwise.
- Custom allows you to add your own type-ins.
- Exact requires the exact word to be inputted, with no suggested type-ins applied.
Since the other two have no customisations, only Custom is worth discussing in detail. When selecting Custom, you'll notice 4 options appear at the bottom of the box:
- + Starts - this is the default option, it allows you to decide what the user must start answers with when typing in. E.g. setting this to "CHINA" means that the user must start their answer with the text "china" to be accepted as an answer.
- + Ends - this is similar to above, except this requires to ending of the typed guess to be something specific. E.g. setting this to "EVEREST" means that the user's guess ends with that, so "everest" and "mounteverest" would both be accepted.
- + Contains - this options allows you to accept guesses containing a specific string of characters. A commmon example of this is "CONGO", which will accept any answer which contains the word "congo" in it somewhere.
- + Regex - this allows the full customisation, it is discussed in complete detail below.
Overall, getting started with type-ins is pretty easy, but actually making type-ins which match against many possible guesses is more useful. For example, you'll never be able to add every possible misspelling of Kyrgyzstan using the first 3 above, for that we need some more powerful tools.
Type-Ins using RegEx
RegEx is short for Regular Expression and refers to a near-universal tool in programming that allows you to pattern match text against cases. We will use a simplified version, since many of the features of RegEx are not useful or available on JetPunk. For example there are characters used to pattern-match on some symbols, but this is useless since the answer-box on JetPunk accepts only alphanumeric input (letters and numbers).
There are 4 different "tokens" that are useful to us:
- Character Classes
- Anchors
- Quantifiers and Alternation
- Capturing Groups
We will go through what each one does, with examples, and then combine them together at the end.
Character Classes
Character Classes define a set of characters to be matched against. These are defined using square brackets [ ]. On their own, they will only match against one letter at a time within the letters between the brackets, called the class.
Examples:- [ABC] - this alone will match any answer which contains an A, B or a C
- F[OEU]D - this will only match when FOD, FED or FUD is entered, since [OEU] only matches against one character (we'll see later with Quantifiers how to increase this)
- [A-G] - you can also use a dash inside to allow it pattern match against any letter between A and G
- [0-7] - this ranging feature also works with numbers
- [AE][FG] - these character classes can be placed next to each other too, this will match when AF, AG, EF or EG is entered
Anchors
Anchors are used to define where we should match against. There are technically 3 anchors that we use:
- ^ - this at the start of a type-in to signify that we want our answer to "Start with" whatever we want
- $ - this goes at the end of a type-in to signify that we want our answer to "End with" whatever we want
- Although this last one is a bit weird, it's actually the absence of ^ and $, which means we want our answer to "Contain" whatever we wish.
You'll notice these 3 options line up with the JetPunk options mentioned above in the Simple Type-Ins section. The downside to using those 3 options is that you can't use any other Regex within them, only plain letters and numbers.
Examples:- ^FOOD - this means we want our answer to start with "FOOD", this is the normal type-in for answers, since it's just matching against you typing in the answer
- ING$ - this will activate whenever anything typed into the text box ends with "ING", e.g. "ing", "thing" or even "abfgvsbfoanfubding"
- INSIDE - this will match any answer which contains the word INSIDE, e.g. "inside" or "whatinsideme" although the second you wouldn't be able to type in 'me' since it will activate the answer against "whatinside"
- ^A[CLN]T - this matches against any answer starting with "act", "alt" or "ant"
I think this gives you an idea of where you could go. It's easy to see that using a ^ and $ in the same answer would be rather pointless in JetPunk's case.
Quantifiers and Alternation
There are 3 main tokens here which are useful for JetPunk and one additional one which is possible to use, but I can't see a use for it particularly.
- + - the plus symbol matches against 1 or more of the preceding token (can be characters or most other tokens)
- ? - the question mark is, suitably, used to match against 0 or 1 of the preceding token. Particularly used to denote optional characters in a type-in
- | - this is the vertical line character called alternation. This acts like a boolean OR operation, meaning it will match the expression before or after the |. It can act on a single group, or on a whole expression
The extra one is *, which matches against 0 or more of the preceding token. Which is plausible to use, but as I mentioned I can't think of a practical use for it.
Examples:- ^FO+D - this means we start with "fo" and then can have any number of o's in the middle, e.g. "fod", "food" and "fooooood" all work
- ^PHILIPP?INES - the original type-in for the Philippines, which allows you to type either 1 or 2 P's, since the second P is optional
- ^MYANMAR|^BURMA - this is using an alternation on the whole expression, and will match an answer when it starts with either "myanmar" or "burma", this is particularly useful for "by borders" quizzes when you run out of type-in boxes (maximum of 10 are allowed)
- ^MAI?N+E - this has an optional "I" and allows 1+ "N"s, so "mane", "maine" and "mainnnnne" will all activate this type-in
- J[OU]+LE - this is a particularly useful combination, since this will allow the token "[OU]" as a whole 1+ times, meaning for this we can match against "JOLE", "JULE", "JOULE" or even "JOUUOUOUOULE"! This comes in handy when you have hard-to-spell words which users may need help with (see next example)
- ^K[IY]RG[A-Z]+STAN - the famous type-in for Kyrgyzstan! This allows any word which starts with K, is followed by an 'I' or 'Y', then an "RG", then the fun bit - any number of letters of the alphabet (at least one) - then finally a "STAN". This means that "KYRGASTAN" and "KIRGVDAUIHDUGAVUDYIHAGDVSTAN" both activate a Kyrgyzstan answer on JetPunk. CAUTION: This "[A-Z]+" combination should be used sparingly, otherwise it may end up accepting answers you did not want it to
Some combinations are not valid, such as ?+ since this logically does not make sense, also +? is valid, but does nothing different to + anyway.
An example of that star token mentioned would be ^MI*NE which allows matches such as "MNE", "MINE" and "MIIIIIINE", since these all contain 0+ I's in the middle. Again this can be done in JetPunk, but I've yet to find a practical example for it.
Capturing Groups
Lastly, capturing groups utilise normal parentheses ( ) to group characters or tokens together. This means you can apply all the previous tools to create any type-in you wish now.
Examples:- ^M(OU|UO)LD - this uses an alternation to match against "ou" OR "uo", so "mould" and "muold" are both accepted. These are used in abundance of type-ins to provide alternative spellings, especially with words ending in "LY"
- ^MAD(MAN)?ME - this uses an optional on an entire capture group, meaning that only answers starting with "madme" and "madmanme" are accepted here
- ^J[AEIOU]T+PUN?K - getting a little more complex here, now allowing the second character to be any vowel, as well as then allowing 1+ T's afterwards and an optional on N. So "jetpunk", "jutttpuk" and "jatpuk" are all valid for this type-in
- ^(SAINT|ST)?H[AE]R+[AEIOL]+S+[EO]N - this looks rather complex, so let's break it down into parts:
- ^(SAINT|ST)? - this is a particularly useful combination for answer which may or may not have a prefix, this will accept any answer that start with "saint" OR "st", or it will accept if this is ignored entirely too thanks to the optional outside the capture group.
- H[AE]R+ - this part requires a "h" followed be a single "a" or "e", then followed by at least one "r".
- [AEIOL]+S+[EO]N - this final part takes at least one letter from "A", "E", "I", "O", "L" (i.e. can be any number of these in combination, as long as there's at least one of one of them), followed by 1+ S's, then a single E or O and finally an N.
Possible matches to that last example are "harasen", "st heroileaoson" or perhaps "saint harrrrrroioieoloieaesssssen".
As you can see, in combination these can be very powerful tools which can provide very useful type-ins for for quiz-makers and quiz-takers.
What's the point?
The point of type-ins is to provide an easier way to match against possible user answers. Yes, it's possible to just type every possible spelling somebody may input as the answer, but what if you miss somebody's way of misspelling? And why do that when we can simplify them?
If we go back to our example of Kyrgyzstan:
^K[IY]RG[A-Z]+STAN
This type-in does wonders since nearly everybody spells Kyrgyzstan incorrectly in a different way at some point. This type-in was made by stripping it back to what it needs to be the word Kyrgyzstan, which means it needs to start with K, then either I or Y followed by RG. We also want it to end with STAN, which all gets combined to produce the type-in shown above.
Finally, many users appreciate when a quizmaker puts effort into type-ins, since this makes it a more pleasant quiz to take, especially when some of the answers come from other languages or can be simply strange in spelling or formation.
Also, if you'd like to explore RegEx further outside of JetPunk to practice or otherwise, RegExr is an excellent tool for getting to grips with all the terminology and features. It is also useful for testing the more complicated type-ins!
I mean when you do a country quiz and its on Europe and you want Cyprus or Azerbaijan already there so no one complains
Simply switch the design mode in step 4 to "manual", and then options to add rows and columns to each block appear, so you can add a new row, type in the pre-filled answer, and then style that row / cell however you'd like (e.g. fill it grey).
[I would know, I was born there ;)]
It was very useful for me ^^
Therefore * still doesn't have a use.
Like if to accept both "mad cow" and "cow mad".
Is (COWMAD|MADCOW) the only option?
word that starts with n
When I try to do that, just typing n enters it in correctly. How do I fix it?
^N[A-Z][A-Z][A-Z][A-Z]
However, this would allow "NNNNN" as a word, which may not be what you want.
Normally you can't get an answer by just putting a space. However if you put * after every letter in your answer, once the quiztaker puts a space (just " ") it will fill in the answer. I've only done this on a preview, I don't know if this works on the real quiz.
The answer is Road No. 5, Jubilee Hills (The source has it this way, so this is how it will show up).
I want Road No. 5 to work. I also want Road 5 to work. I also want Road Number 5 to work.
I ended up doing ^ROAD[A-Z]*(FIVE|5)
I am allowing someone to put anything between road and 5.
Using ? would mean they are limited to 2 letters, not allowing them to do Road Number 5. Using + Means they MUST put a letter there.
* is the solution!
^ROAD(NO|NUMBER)?(FIVE|5)
Since this prevents many variations being allowed
It's also technically faster to write.
Your solution is perfectly plausible. I'm going to keep my solution though since I think it makes the quiztaking experience easier. I don't know what variations someone would even try that would trigger this.
I think it could be useful in a case where there are many optional words in the middle of a type in.
If that makes sense.
Im Having Problems. How do i make a quiz with many answers to one question? Im trying to make one, but i can add that many type ins. So Help Pls.
Thank You
Alternatively, you can put more than 1 typein in a specific line. If you add a regex line, you can add multiple typeins using |. E.g. ^Alpha|^Beta|^Gamma
Ok Say I wanted to make a quiz that is country by letter but there is none by that letter. How do i make it show up that there is none?
To add a link to another page / website etc... Simply use the following HTML, removing the space after the "<":
< a href="URL">TEXT< /a>
Replacing URL and TEXT with whatever you want the link to say and go to.
If there is a * present, parenthesis serve as square brackets. (PA)*L accepts L, AAL, and PAPAL.
If a + present, the inside information can be repeated. (PA)+L accepts PAPAL and PAL, but not AAL.
Otherwise, they are ignored. (PA)L only accepts PAL.
However, if there is a hyphen when there is no + or * like (A-Z)STAN, there doesn't seem to be any text that works. How does that function?
Parentheses are just subsets of input data used to separate sections of the regex.
Square brackets however are character classes and have special functions to allow them to have different behaviours. E.g. - for character ranges, [^d] for negation etc...
My source says 8 in hindi is Aaath. Another says Aath, I also want Ath to be accepted. So ^AA*T (i also allow the omission of the h. So is this finally a use for *!!?!?!?
- Wie kann ich bei einem Quiz bei manchen „Hints“ zwei Antwortmöglichkeiten, die jedoch beide genannt werden müssen, bei anderen jedoch nur eine einstellen?
- Wie kann ich es einstellen, dass „Hints“ nacheinander beantwortet werden müssen und gleichzeitig nicht andere „Hints“ beantwortet werden, welche den gleichen Begriff als Antwort haben?
First, you can only have a single answer column in Step 2, so you must put all answers separately in this area.
In Step 4, you can enable "Manual" design mode at the top of the page, and on the right-hand side will appear several options for adding/removing rows, columns and blocks (like whole groups of columns).
This will allow you to add cells to whatever design you wish. Afterwards, select a cell and choose an answer or hint using the "Manual Cell Options" at the top of the page.